Skhokho 💪 🧑🏽💻 🔥
Python GPT Neo
In this note, there is information of how to set up and use the Python GPT-Neo
Pre-Requisites
Get a digital ocean droplet to work from: https://m.do.co/c/7d9a2c75356d
#Create new user
adduser skolo
#Add user to sudo group
usermod -aG sudo skolo
#Copy ssh keys from root to new user
rsync --archive --chown=skolo:skolo ~/.ssh /home/skolo
#Log out and log in to the new user
#Follow this Flask Tutorial
#Watch Skolo Online Video on Youtube as well to fill in the blanks
Step 1: Install PyTorch
Website link: https://pytorch.org/
Linux pip command:
pip install torch==1.10.2+cpu torchvision==0.11.3+cpu torchaudio==0.10.2+cpu -f https://download.pytorch.org/whl/cpu/torch_stable.html
Step 2: Install Transformers
Website link: https://huggingface.co/docs/transformers/index
pip command: pip install transformers
Step 3: Create a python file
Contents of the file
#import pipeline from transformers
from transformers import pipeline
#Generate Text Pipeline
generator = pipeline('text-generation', model='EleutherAI/gpt-neo-2.7B')
#create a prompt
prompt = "The amazing spiderman"
#Get the response from Model
res = generator(prompt, max_length=100, do_sample=True, temperature=0.8)
# Printing the output to a text name as generated_text
print(res[0]['generated_text'])
#save the generated text in to a file
with open('gpttext.txt', 'w') as f:
f.writelines(res[0]['generated_text'])
What more we van do with Pipeline
Pipeline models: https://huggingface.co/models?pipeline_tag=text-generation
Text Classification
classifier = pipeline('sentiment-analysis')
classifier('Enter the text here you would like to classify')
#the response will be negative or positive, with a percentage indication
#you can also enter a list and the response will be with a list
#you can also enter custom labels as so:
classifier = ('Enter the text here that you want to classify', candidate_labels=['Education', ‘Politics’, ‘Business’])
Text Generation
generator = pipeline('text-generation', model='distilgpt2')
generator('What is the meaning of life', max_length=30, num_return_sequences=3)
Language Translation
translator = pipeline('translation', model='Helsinki-NLP/opus-mt-zh-en')
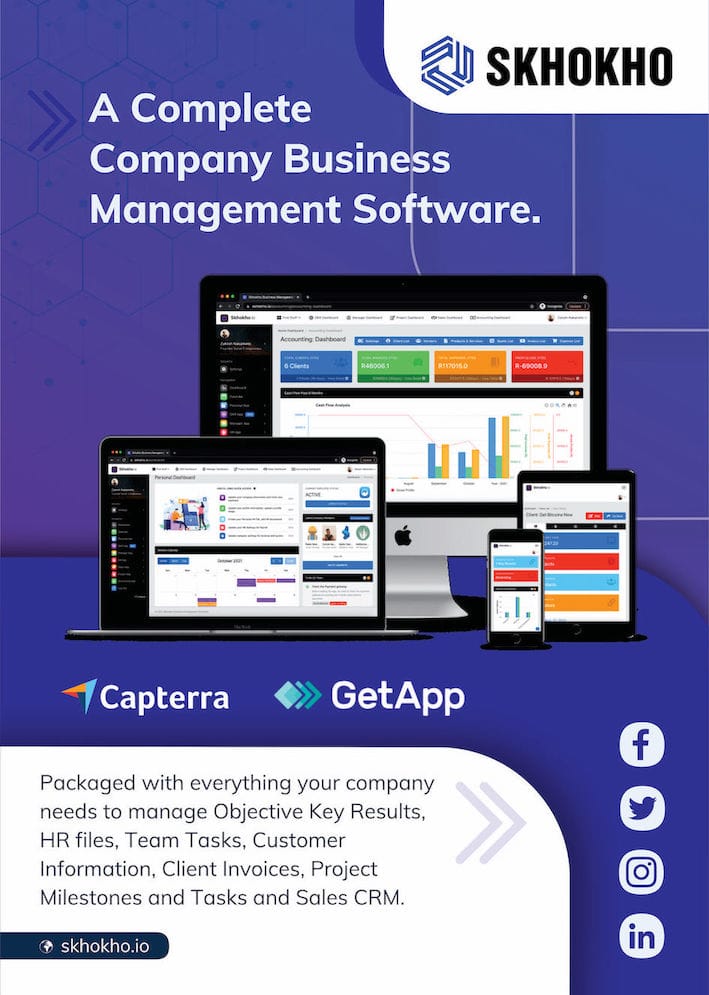